pug 是一个模板引擎。 模板引擎用于消除ag捕鱼王app官网的服务器代码与 html 的混乱,将字符串连接到现有的 html 模板。 pug 是一个非常强大的模板引擎,它具有多种功能,例如 filters, includes, inheritance, interpolation等。这方面的内容很多。
要将 pug 与 koa 一起使用,我们需要使用以下命令安装它。
$ npm install --save pug koa-pug
安装 pug 后,将其设置为应用程序的模板引擎。 将以下代码添加到我们的 app.js 文件中。
var koa = require('koa');
var router = require('koa-router');
var app = new koa();
var pug = require('koa-pug');
var pug = new pug({
viewpath: './views',
basedir: './views',
app: app //等价于 app.use(pug)
});
var _ = router(); // 实例化路由器
_.get('/hello', async (ctx,next) => { // 定义路由
await ctx.render('first_view');
});
app.use(_.routes()); // 使用由 router 定义的路由
app.listen(3000);
现在,创建一个名为 views 的新目录。 在该目录中,创建一个名为 first_view.pug 的文件,并在其中输入以下数据。
doctype html
html
head
title hello pug
body
p.greetings#people hello 迹忆客!
运行程序,显示结果如下
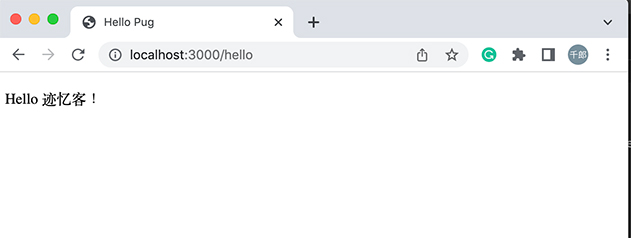
pug 所做的是,它将这个看起来非常简单的标记转换为 html。 我们不需要跟踪关闭我们的标签,不需要使用 class 和 id 关键字,而是使用 .
和 #
来定义它们。 上面的代码首先被转换为
hello pug
hello 迹忆客!
pug 能够做的不仅仅是简化 html 标记。 让我们探索一下 pug 的一些功能。
简单标签
标签根据它们的缩进嵌套。 就像上面的例子一样,</code> 在 <code><head></code> 标记内缩进,所以它在里面。 但是,<code><body></code> 标签在同一个缩进上,因此它是 <code><head></code> 标签的兄弟。</p>
<p>我们不需要关闭标签。 一旦 pug 在同一缩进级别或外部缩进级别上遇到下一个标签,它就会为我们关闭标签。</p>
<p>有三种方法可以将文本放入标签内</p>
<ul>
<li>空格分隔<pre><code class="language-html">h1 welcome to pug
</code></pre>
</li>
<li>管道文本<pre><code class="language-html">div
| to insert multiline text,
| you can use the pipe operator.
</code></pre>
</li>
<li>文本块<pre><code class="language-html">div.
but that gets tedious if you have a lot of text.
you can use "." at the end of tag to denote block of text.
to put tags inside this block, simply enter tag in a new line and
indent it accordingly.
</code></pre>
</li>
</ul>
<h3 id="注释">注释</h3>
<p>pug 使用与 javascript(//) 相同的语法来创建注释。 这些注释被转换为 html 注释(<code></code>)。 例如,</p>
<pre><code class="language-javascript">// 这是一个 pug 注释
</code></pre>
<p>此注释被转换为</p>
<pre><code class="language-html">
</code></pre>
<h3 id="属性">属性</h3>
<p>为了定义属性,我们在括号中使用逗号分隔的属性列表。 类和 id 属性有特殊的表示。 以下代码行涵盖了为给定的 html 标记定义属性、类和 id。</p>
<pre><code class="language-html">div.container.column.main#division(width = "100",height = "100")
</code></pre>
<p>这行代码,被转换为</p>
<pre><code class="language-html"><div class = "container column main" id = "division" width = "100" height = "100"></div>
</code></pre>
<h3 id="将值传递给模板">将值传递给模板</h3>
<p>当我们渲染一个 pug 模板时,我们实际上可以从我们的路由处理程序中传递一个值,然后我们可以在我们的模板中使用它。 使用以下代码创建一个新的路由处理程序。</p>
<pre><code class="language-javascript">var koa = require('koa');
var router = require('koa-router');
var app = new koa();
var pug = require('koa-pug');
var pug = new pug({
viewpath: './views',
basedir: './views',
app: app //等价于 app.use(pug)
});
var _ = router(); // 实例化路由器
_.get('/hello', async (ctx,next) => { // 定义路由
await ctx.render('first_view');
});
_.get("/dynamic_view", async (ctx,next) => {
await ctx.render('dynamic', {
name: "迹忆客",
website: "jiyik.com"
url:"https://www.jiyik.com"
});
})
app.use(_.routes()); // 使用由 router 定义的路由
app.listen(3000);
</code></pre>
<p>然后,使用以下代码在 views 目录中创建一个名为 <code>dynamic.pug</code> 的新视图文件。</p>
<pre><code class="language-html">html
head
title #{name}
body
h1 #{name}
a(href = url) url
</code></pre>
<p>在浏览器中打开 <strong>localhost:3000/dynamic</strong> ,以下是输出。</p>
<p><img src="/uploads/220326/i_20220326203644edfba4.jpg" alt="koa 动态模板"></p>
<h3 id="条件">条件</h3>
<p>我们也可以使用条件语句和循环结构。 考虑这个实际示例,如果用户已登录,我们希望显示“hi, user”,如果没有,则我们希望向他显示“登录/注册”链接。 为此,我们可以定义一个简单的模板,例如</p>
<pre><code class="language-html">html
head
title simple template
body
if(user)
h1 hi, #{user.name}
else
a(href = "/sign_up") sign up
</code></pre>
<p>当我们使用我们的路由渲染它时,如果我们传递一个对象,像下面这样</p>
<pre><code class="language-javascript">ctx.render('/dynamic',{user:
{name: "ayush", age: "20"}
});
</code></pre>
<p>它会显示一条消息,显示 hi, ayush。 但是,如果我们不传递任何对象或传递一个没有用户密钥的对象,那么我们将获得一个注册链接。</p>
<h3 id="include-和-组件">include 和 组件</h3>
<p>pug 提供了一种非常直观的方式来为网页创建组件。 例如,如果你看到一个新闻网站,带有徽标和类别的标题始终是固定的。 我们可以使用 <code>include</code>,而不是将其复制到每个视图中去。 以下示例显示了我们如何使用 include</p>
<p>使用以下代码创建三个视图</p>
<blockquote class="alert alert-info">
<p><strong><code>header.pug</code></strong></p>
<pre><code class="language-html">div.header.
i'm the header for this website.
</code></pre>
</blockquote>
<blockquote class="alert alert-info">
<p><strong><code>content.pug</code></strong></p>
<pre><code class="language-html">html
head
title simple template
body
include ./header.pug
h3 i'm the main content
include ./footer.pug
</code></pre>
</blockquote>
<blockquote class="alert alert-info">
<p><strong><code>footer.pug</code></strong></p>
<pre><code class="language-html">div.footer.
i'm the footer for this website.
</code></pre>
</blockquote>
<p>为此创建一个路由,如下所示。</p>
<pre><code class="language-javascript">var koa = require('koa');
var router = require('koa-router');
var app = new koa();
var pug = require('koa-pug');
var pug = new pug({
viewpath: './views',
basedir: './views',
app: app //等价于 app.use(pug)
});
var _ = router(); // 实例化路由器
_.get('/hello', async (ctx,next) => { // 定义路由
await ctx.render('first_view');
});
_.get("/dynamic_view", async (ctx,next) => {
await ctx.render('dynamic', {
name: "迹忆客",
url:"https://www.jiyik.com"
});
})
_.get('/components', async (ctx,next) => {
await ctx.render('content');
});
app.use(_.routes()); // 使用由 router 定义的路由
app.listen(3000);
</code></pre>
<p>访问 <strong>localhost:3000/components</strong> ,我们应该会看到如下输出内容。</p>
<p><img src="/uploads/220326/i_202203262047194b2335.jpg" alt="koa 模板include"></p>
<p><strong>include</strong> 也可用于包含纯文本、css 和 javascript。</p>
<p>pug 还有许多其他功能。 但是,这些超出了本教程的范围。 大家可以 <a href="https://jade-lang.com/" target="_blank">点击这</a> 进一步探索 pug。</p></div>
</div>
</div>
<div class="previous-next-links">
<div class="previous-design-link">
<a href="/w/koa/koa-cascading">
<span class="iconfont" style="color: #387a9f;"></span>
</a>
<a href="/w/koa/koa-cascading" rel="prev" title="koa.js cascading 级联">koa.js cascading 级联</a>
</div>
<div class="next-design-link">
<a href="/w/koa/koa-form" rel="next" title="koa.js 表单 - form">koa.js 表单 - form</a>
<a href="/w/koa/koa-form">
<span class="iconfont" style="color: #387a9f;"></span>
</a>
</div>
</div>
</div>
<div style="margin-bottom:10px;">
<style>
.responsive_ad1 { display:inline-block;}
@media (max-width: 500px) { .responsive_ad1 { display: none; } }
@media(min-width: 800px) { .responsive_ad1 { width: 840px; height: 100px; } }
</style>
<script async src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js?client=ca-pub-9072260435000715"
crossorigin="anonymous"></script>
<ins class="adsbygoogle responsive_ad1"
style="min-width:500px;max-width:840px;width:100%;height:100px;"
data-ad-client="ca-pub-9072260435000715"
data-ad-slot="8089067372"
data-full-width-responsive="true"></ins>
<script>
(adsbygoogle = window.adsbygoogle || []).push({});
</script>
</div>
<div class="jyk_question_comments">
<h2 class="">
<div class="altblock" data-expand="0">
<i style="font-size:24px;padding-right:0px;" class="iconfont" aria-hidden="true"></i>
</div>
<span class="mw-headline" id="qa_headline">查看笔记</span>
</h2>
</div>
<div class="leave-message" id="leave-message" style="display:none;"></div>
</section>
</main>
<aside class="question_right mt15">
<div class="right_nav">
<h2>
<span class="iconfont"></span>分类导航
</h2>
<ul class="two_nav">
<li class="two_nav_item">
<a href="javascript:;" >服务端</a>
<ul class="three_nav">
<li><a href="/w/ba4j11165">正则表达式</a></li>
<li><a href="/w/perl">perl 教程</a></li>
<li><a href="/w/hjsp35191">php-正则</a></li>
<li><a href="/w/java">java 教程</a></li>
<li><a href="/w/php">php教程</a></li>
<li><a href="/w/php7">php7 教程</a></li>
<li><a href="/w/python">python 教程</a></li>
<li><a href="/w/lua">lua 教程</a></li>
<li><a href="/w/python3">python 3 教程</a></li>
<li><a href="/w/go">go 教程</a></li>
<li><a href="/w/graphql">graphql 教程</a></li>
<li><a href="/w/node">nodejs 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >web端</a>
<ul class="three_nav">
<li><a href="/w/css3">css3 教程</a></li>
<li><a href="/w/html">html 教程</a></li>
<li><a href="/w/html5">html5 教程</a></li>
<li><a href="/w/css">css 教程</a></li>
<li><a href="/w/sass">sass 教程</a></li>
<li><a href="/w/less">less 教程</a></li>
<li><a href="/w/wp">wordpress</a></li>
<li><a href="/w/svg">svg 教程</a></li>
<li><a href="/w/xml">xml教程</a></li>
<li><a href="/w/bootstrap5">bootstrap 5 教程</a></li>
<li><a href="/w/bootstrap4">bootstrap 4 教程</a></li>
<li><a href="/w/bootstrap3">bootstrap 3 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >javascript</a>
<ul class="three_nav">
<li><a href="/w/ts">typescript 教程</a></li>
<li><a href="/w/popperjs">popper.js 中文教程</a></li>
<li><a href="/w/underscore">underscore.js 中文教程</a></li>
<li><a href="/w/nextjs">next.js 中文教程</a></li>
<li><a href="/w/chartjs">chart.js 中文教程</a></li>
<li><a href="/w/dc">dc.js 中文教程</a></li>
<li><a href="/w/javascript">javascript 教程</a></li>
<li><a href="/w/recoil">recoil.js</a></li>
<li><a href="/w/jquery">jquery 教程</a></li>
<li><a href="/w/es6">es6 教程</a></li>
<li><a href="/w/angularjs">angularjs 教程</a></li>
<li><a href="/w/webpack">webpack 教程</a></li>
<li><a href="/w/pm2">pm2 教程</a></li>
<li><a href="/w/vue">vue.js 教程</a></li>
<li><a href="/w/vue3">vue3 教程</a></li>
<li><a href="/w/d3">d3.js 教程</a></li>
<li><a href="/w/echarts">echarts 教程</a></li>
<li><a href="/w/json">json 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >数据库</a>
<ul class="three_nav">
<li><a href="/w/zqxs17511">redis教程</a></li>
<li><a href="/w/mongodb">mongodb 教程</a></li>
<li><a href="/w/memcached">memcached 教程</a></li>
<li><a href="/w/sql">sql 教程</a></li>
<li><a href="/w/sqlite">sqlite 教程</a></li>
<li><a href="/w/postgresql">postgresql 教程</a></li>
<li><a href="/w/es">elastic search</a></li>
<li><a href="/w/db2">db2 教程</a></li>
<li><a href="/w/mysql">mysql 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >java 技术</a>
<ul class="three_nav">
<li><a href="/w/spring">spring 框架</a></li>
<li><a href="/w/maven">maven 中文教程</a></li>
<li><a href="/w/mybatis">mybatis 中文教程</a></li>
<li><a href="/w/spboot">spring boot 中文教程</a></li>
<li><a href="/w/sporm">spring boot orm</a></li>
<li><a href="/w/hibernate">hibernate 教程</a></li>
<li><a href="/w/struts">struts 教程</a></li>
<li><a href="/w/log4j">log4j 教程</a></li>
<li><a href="/w/slf4j">slf4j 教程</a></li>
<li><a href="/w/mapstruct">mapstruct 教程</a></li>
<li><a href="/w/java8">java8 教程</a></li>
<li><a href="/w/java11">java 11 教程</a></li>
<li><a href="/w/gradle">gradle</a></li>
<li><a href="/w/neo4j">neo4j 教程</a></li>
<li><a href="/w/guava">guava 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >后端框架</a>
<ul class="three_nav">
<li><a href="/w/laravel">laravel 教程</a></li>
<li><a href="/w/nest">nest.js 中文教程</a></li>
<li><a href="/w/koa">koa.js 中文教程</a></li>
<li><a href="/w/express">express.js 中文教程</a></li>
<li><a href="/w/sequelize">sequelize 中文教程</a></li>
<li><a href="/w/hapi">hapi 中文教程</a></li>
<li><a href="/w/junit">junit 教程</a></li>
<li><a href="/w/scrapy">scrapy 教程</a></li>
<li><a href="/w/gin">gin 教程</a></li>
<li><a href="/w/gorm">gorm 教程</a></li>
<li><a href="/w/django">django 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >数据分析</a>
<ul class="three_nav">
<li><a href="/w/zk">zookeeper</a></li>
<li><a href="/w/numpy">numpy 教程</a></li>
<li><a href="/w/scipy">scipy 教程</a></li>
<li><a href="/w/matplotlib">matplotlib 教程</a></li>
<li><a href="/w/pandas">pandas 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >开发工具</a>
<ul class="three_nav">
<li><a href="/w/docker">docker 教程</a></li>
<li><a href="/w/markdown">markdown 教程</a></li>
<li><a href="/w/k8s">k8s 教程</a></li>
<li><a href="/w/flutter">flutter 教程</a></li>
<li><a href="/w/makefile">makefile</a></li>
<li><a href="/w/tkinter">tkinter 教程</a></li>
<li><a href="/w/matlab">matlab 教程</a></li>
<li><a href="/w/git">git 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >网络</a>
<ul class="three_nav">
<li><a href="/w/website">网站开发教程</a></li>
<li><a href="/w/soap">soap</a></li>
<li><a href="/w/http">http 教程</a></li>
<li><a href="/w/nginx">nginx 教程</a></li>
<li><a href="/w/ipv4">ipv4 教程</a></li>
<li><a href="/w/ipv6">ipv6 教程</a></li>
</ul>
</li>
<li class="two_nav_item">
<a href="javascript:;" >编程手册</a>
<ul class="three_nav">
<li><a href="/w/html-ref">html 参考手册</a></li>
<li><a href="/w/jquery/jquery-ref-selectors">jquery 参考手册</a></li>
</ul>
</li>
</ul>
</div>
<div class="mt15">
<script async src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js?client=ca-pub-9072260435000715"
crossorigin="anonymous"></script>
<ins class="adsbygoogle"
style="display:block"
data-ad-client="ca-pub-9072260435000715"
data-ad-slot="8124726399"
data-ad-format="auto"
data-full-width-responsive="true"></ins>
<script>
(adsbygoogle = window.adsbygoogle || []).push({});
</script>
</div>
</aside>
</div>
<script type="text/javascript">
$(function () {
$(".two_li .two_li_txt").click(function() {
$(this).find("i").toggleclass('on');
$(this).next().slidetoggle();
})
$(".altblock").click(function (e) {
if($(this).data('expand') == 0) {
$("#leave-message").show();
$(this).data('expand',1);
$(this).find("i").html('');
}else{
$("#leave-message").hide();
$(this).data('expand',0);
$(this).find("i").html('')
}
});
hljs.inithighlighting();
// 手机端二级按钮
$(".ej_btn").click(function(event) {
$(".question_left").animate({left: 0}, 500);
});
$.ajax({
url: '/w/count/a/koa-template',
type: 'post',
datatype: 'json',
success: function (res) {
console.log(res);
}
})
});
onmpwm({
id:'leave-message',
aid:'koa-template',
width:"100%",
headtext:"笔记",
welcometext:"欢迎留下您的杰作",
title:'koa.js 模板',
type:2,
highlight:true, // 代码是否高亮显示
// placeholder:'我要记笔记...'
});
</script>
<footer>
<div class="foot_wrap">
<dl>
<dt>网站导航</dt>
<dd><a href="/tm/xwzj" target="_blank">编程</a></dd>
<dd><a href="/w" target="_blank">教程</a></dd>
<dd><a href="/q" target="_blank">题库</a></dd>
<dd><a href="/tm/qwzt" target="_blank">随笔</a></dd>
<dd><a href="/tm/liuyan" target="_blank">留言</a></dd>
</dl>
<dl class="tutorial">
<dt>教程更新</dt>
<dd><a href="/w/nextjs/nextjs-impreative-routing" target="_blank">next.js 命令式(imperative)路由</a></dd>
<dd><a href="/w/nextjs/nextjs-dynamic-routes" target="_blank">next.js 动态路由</a></dd>
<dd><a href="/w/nextjs/nextjs-pre-rendering" target="_blank">next.js 预渲染 pre rendering</a></dd>
<dd><a href="/w/nextjs/nextjs-global-css-support" target="_blank">next.js 添加全局内置 css 样式</a></dd>
<dd><a href="/w/nextjs/nextjs-css-support" target="_blank">next.js 内置对 css 的支持</a></dd>
<dd><a href="/w/nextjs/nextjs-meta-data" target="_blank">next.js 元数据 - meta data</a></dd>
<dd><a href="/w/nextjs/nextjs-static-serving" target="_blank">next.js 静态文件服务</a></dd>
</dl>
<dl>
<dt>站点信息</dt>
<dd><a href="http://mail.qq.com/cgi-bin/qm_share?t=qm_mailme&email=pa0ocagpcasicg18tu0sx1nr" target="_blank" rel="nofollow">意见反馈</a></dd>
<dd><a href="/disclaimer" target="_blank" rel="nofollow">免责声明</a></dd>
<dd><a href="/tm/zj" target="_blank">足迹</a></dd>
</dl>
<div class="foot_share">
<img width="140" src="/static/jiyi/v3/img/ew.jpg" alt="小程序:迹忆计算机教程">
<p>扫一扫,随时随地看教程</p>
</div>
</div>
<div class="foot_ag捕鱼王app官网 copyright">design by <a href="/" target="_blank">迹忆客</a> <a href="https://beian.miit.gov.cn" target="_blank" rel="nofollow">冀icp备15007416号-2</a></div>
</footer>
<div class="dimmer"></div>
<div class="off-canvas">
<div class="canvas-close">
<i></i>
</div>
<div class="mobile-menu d-block d-xl-none d-lg-none">
</div>
</div>
<div class="right_fixed">
<a class="return_top iconfont" href="javascript:;" title="返回顶部" style="display:inline-block;"></a>
<a class="ew iconfont" href="javascript:;" title="小程序"></a>
</div>
<div id="bottom-qrcode" class="min_pro" >
<div class="text">
<div class="download-text c-font-big">扫码一下</div>
<div class="c-font-normal c-color-gray2">查看教程更方便</div>
</div>
<img class="qrcode" src="/static/jiyi/v3/img/ew.jpg">
</div>
<style>
.right_fixed{position: fixed;right:1%;bottom: 25%;width:58px;border:1px solid #eee;font-size:24px;}
.right_fixed a{width:56px;height:56px;display: inline-block;text-align:center;font-size:30px;line-height:56px;background-color:#fff;padding-right:0;color:#2a7e9f;}
.right_fixed .return_top{border-bottom: 1px solid #eee;display: none;}
.min_pro{bottom:25%;height: 115px;width:259px;padding: 10px;margin: 0;right: 86px;position: fixed;box-shadow: 0 2px 10px 0 rgb(0 0 0 / 10%);border-radius:12px;background-color: #fff;display:none;}
.min_pro .text{text-align: left;margin-top: 24px;margin-left: 16px;font-size:14px;}
/* .min_pro .text::after{content:""; display: block; width: 0; height: 0; border-style: solid; border-color: transparent transparent transparent #154079; border-width: 6px; position: absolute; bottom: 12%; right: -12px; margin-left: -6px;box-shadow: 0 2px 10px 0 rgb(0 0 0 / 10%);} */
.min_pro img{position: absolute;width: 100px;height: 100px;top: 7px;right: 16px}
</style>
<script>
$(window).scroll(function(){
var _top = $(document).scrolltop();
if(_top > 200){
$(".return_top").fadein();
}else{
$(".return_top").fadeout();
}
})
$(".return_top").click(function(){
$('html,body').animate({scrolltop:0}, 100);
return false;
})
$(".ew").hover(function(){
$("#bottom-qrcode").show();
},function(){
$("#bottom-qrcode").hide();
});
</script>
<script async src="https://www.googletagmanager.com/gtag/js?id=g-hh7bmnwdqh"></script>
<script>
window.datalayer = window.datalayer || [];
function gtag(){datalayer.push(arguments);}
gtag('js', new date());
gtag('config', 'g-hh7bmnwdqh');
</script>
<script>
var _hmt = _hmt || [];
(function() {
var hm = document.createelement("script");
hm.src = "https://hm.baidu.com/hm.js?96a3b22d959b1d2caea488dcccd275d2";
var s = document.getelementsbytagname("script")[0];
s.parentnode.insertbefore(hm, s);
})();
</script>
<script>
(function(){
var el = document.createelement("script");
el.src = "https://lf1-cdn-tos.bytegoofy.com/goofy/ttzz/push.js?86472f8d504d87358aff2b46abbe30ef4b42c9ccd9aa6e93a72da00905da80838538161d7ba7d69bfe7b93bc414b7758ce8074c9b6dd4529067719a8bf38e94a";
el.id = "ttzz";
var s = document.getelementsbytagname("script")[0];
s.parentnode.insertbefore(el, s);
})(window)
</script>
</body>
</html>